In this Razor tutorial, we will take a quick tour of the Razor View Engine in ASP.NET Core MVC. The Razor makes it easier to embed the C# code inside the HTML, thus providing the ability to generate the dynamic response. In this tutorial, we will explore the Razor syntax.
Table of Contents
What is View Engine
The View Engine is responsible for producing an HTML response when invoked by the Controller Action method.
The Controller Action methods can return various types of responses, which are collectively called as Action Results. The ViewResult is the ActionResult which produces the HTML Response.
The ViewResults are produced by the View Engine. When the Controller Action method invokes the view() or PartivalView(), it invokes the View Engine, which produces the HTML Response.
What is Razor View Engine
The Razor View Engine is the default View Engine for the ASP.NET Core apps. It looks for Razor markup in the View File and parses it and produces the HTML response.
The Razor Markup
The Controller in MVC invokes the View by passing the data to render. The Views must have the ability to process the data and generate a response. This is done using the Razor markup, which allows us to use C# code in an HTML file. The Razor View Engine process these markups to generate the HTML.
The files containing Razor markup generally have a .cshtml file extension.
The Razor syntax is shorter and simpler and easy to learn as it uses the C# or visual basic. The Visual Studio IntelliSense support also helps with Razor syntax.
Example Project
To know how Razor works create an Empty Project and add MVC Middlewares and services. You can refer to the tutorial Building ASP.NET Core Application.
You can download the source code from the GitHub. The initial Code is in the folder Start and the completed code in the folder Razor
Goto the index method of the HomeController
1 2 3 4 5 6 | public IActionResult Index() { return View(); } |
Open the index.cshtml and copy the following code.
1 2 3 | <h1>Razor Example</h1> |
Run the app and you should see Razor Example in the browser.
Now, go to the Models Folder and create a new class Customer.cs.
1 2 3 4 5 6 7 8 9 10 | namespace MVCCoreApp.Models { public class Customer { public string name { get; set; } public string address { get; set; } } } |
The Razor Syntax
The Razor uses the @ symbol to transition from HTML markup to the C# code.The following are the two ways, by which you can achieve the transitions.
- Using Razor code expressions
- Using Razor code blocks.
These expressions are evaluated by the Razor View Engine and written to the response.
Razor Code blocks
The Razor code blocks start with @ symbol followed by curly braces. You can use code blocks anywhere in the markup.
A Razor code block can be used manipulate a model, declare variables, and set local properties on a view etc. However, you should not use it for business logic.
Now, Open the index.html and copy the following code.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <h3>Code Block</h3> @{ var greeting = "Welcome to our site!"; var weekDay = DateTime.Now.DayOfWeek; } @{ var cust = new MVCCoreApp.Models.Customer() { name = "Rahul Dravid", address = "Bangalore" }; } |
First, we have created a Razor code block beginning with a @ symbol and { } curly brackets. Inside the Curly brackets, we have a regular C# code, which declares a greeting & weekDay Variable.
The Second Razor Code block creates a cust variable, which is a new instance of the Customer model.
Razor Code Expressions
The Razor Code expressions start with @ and followed by C# code. The Code expression can be either Implicit or Explicit.
Implicit Razor Expressions
Implicit Razor expressions start with @ followed by C# code like the one mentioned above.
In the following example, the code @greeting, @DateTime.Now, @WeekDay are treated as Implicit Razor expressions. Space is not allowed in the Code expression, as it is used to identify the end of the expression. The expressions are evaluated by the Razor View engine and the result is inserted in their place.
1 2 3 4 5 6 7 | <h3>Code Expression</h3> <p>@greeting</p> <p>@DateTime.Now</p> <p>Today is : @WeekDay thank you </p> |
The following Razor code expression displays name and address inside the <p> tag.
1 2 3 4 | <p>Name : @cust.name</p> <p>Address : @cust.address</p> |
Explicit Razor Expressions
Explicit Razor expressions start with @ followed by (). Any content within the () parenthesis is evaluated and rendered to the output.
1 2 3 4 5 6 7 | @{ var ISBNNo = "10001200"; } <p>ISBN : @ISBNNo</p> @*//<p>ISBN@ISBNNo</p> //Does not work*@ <p>ISBN@(ISBNNo)</p> |
IntelliSense support
The image below shows how the Razor markup is used inside an HTML page and the Intellisense support from the Visual Studio.
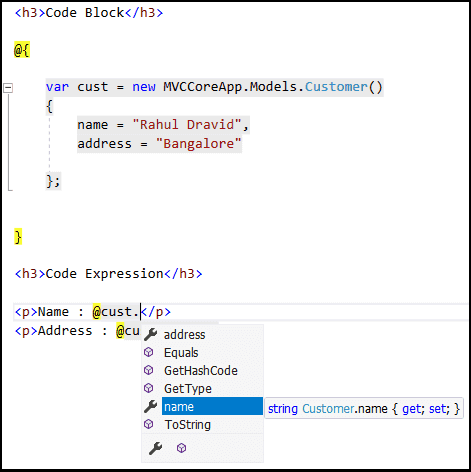
Using Directive
The @using directive works similar to the C# using directive and allows you to import namespaces. The MVCCoreApp.Models can be imported as shown below
1 2 3 | @using MVCCoreApp.Models; |
And then we can simply use var cust = new Customer() instead of var cust = new MVCCoreApp.Models.Customer()
Variable Declaration
The Variables are declared using the var keyword or using the c# data type. The int, float, decimal, bool, DateTime & string keywords can be used to store strings, numbers, and dates, etc.
The variables are inserted directly into a page using @.
1 2 3 4 5 6 7 8 9 10 11 12 | <h3>Variables </h3> <!-- Storing a string --> @{ var message = "Welcome to our website"; } <!-- Storing a date --> @{ DateTime date = DateTime.Now; } <p>@message</p> <p> The current date is @date</p> |
Strings are enclosed in double quotation marks.
To use a double quotation mark inside the string, use a verbatim string literal. The verbatim string is prefixed with the @ symbol and repeat the quotation mark.
1 2 3 4 5 | @{ var helloWorld = @"Hello ""World"""; } <p>@helloWorld</p> |
Similarly, backslash character can be printed using the same technique.
1 2 3 4 5 | @{ var Path = @"C:\Windows\"; }<p> The path is: @Path</p> |
You can print @ in HTML by repeating (Escape) the @ symbol as shown below.
1 2 3 4 5 | @{ var symbol = "You can print @ in html"; } <p>The @@symbol is: @symbol</p> |
The @ in the email address is correctly identifies by the Razor engine and does not to treat the @ as a code delimiter. You do not have to escape it with @.
1 2 3 |
Comments
Use @* *@ to place comments
1 2 3 | @*This is comment*@ |
HTML Elements inside code block
Any HTML Elements inside the Razor code block is correctly identified by the Razor engine as shown below.
1 2 3 4 5 | @{ <p>Hello from the Code block</p> } |
Single line text
You can output the literal values without HTML element by prefixing it with @:
1 2 3 4 5 | @{ @:Hello from the Code block } |
The @: used to define the single line of literal values
Multiline text
For the multiline text use the <text> </text> element
1 2 3 4 5 | @{ <text>Hello from the multiline text </text> } |
Conditional Statements
The Razor engine is able to process conditional statements like if & Switch statement.
If and Else Conditions
If Condition is used to render a section based on a condition as shown below.
1 2 3 4 5 6 7 8 9 10 11 12 | @{int value = 200;} @if (value > 100) { <p>Value is greater than 100.</p> } else { <p>Value is less than 100.</p> } |
Or you can use the following code
1 2 3 4 5 6 7 8 9 10 11 12 13 | @{ var value = 200; if (value > 100) { <p>The value is greater than 100 </p> } else { <p>This value is less than 100.</p> } } |
An If else if else block
1 2 3 4 5 6 7 8 9 10 11 | @if (SomeCondition) { } else if(SomeOtherCondition) { } else { } |
Switch Statements
A switch statement can insert a section into HTML based on a number of conditions.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | @switch (value) { case 0: @: value is Zero break; case 100: <p>Value is 100 </p> break; case 200: <p>Value is @value </p> break; case 300: <text>Value is 300</text> break; default: <p>Invalid Value </p> break; } |
Loops
Foreach loop
The loops are used to repeat a code block for
1 2 3 4 5 6 | @for (int i = 0; i < 5; i++) { <span> @i </span> } |
The best use case of a foreach loop is to loop through a collection object and display the result inside table
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | @{ var custList = new List<Customer>() { new Customer() { name = "Rahul", address = "Bangalore" }, new Customer() { name = "Sachin", address = "Mumbai" } }; } <table> <thead> <tr><td>Name</td><td>Address</td></tr> </thead> @foreach (Customer custvar in custList) { <tr> <td>@custvar.name</td> <td>@custvar.address</td> </tr> } </table> |
While loop
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | <h3>While loop</h3> @{ var r = 0; while (r < 5) { r += 1; <span> @r</span> } } @{ var s = 0; } @while (s < 5) { s += 1; <span> @s</span> } } |
HTML Encoding
All the Razor expressions are automatically HTML Encoded.
For Example
1 2 3 4 5 6 | @{ string encodedMessage = "<script>alert('You are under cross-site script injection attack');</script>"; } <span>@encodedMessage </span> |
The above code will not result in an alert box. Instead, you will see the “<script>alert(‘You are under cross-site script injection attack’);</script>” printed in the browser.
The Razor automatically encodes the < to < and > to >
If you look at the generated HTML Response you will see this
<span><script>alert(' You are under cross-site script injection attack') ;</script> </span>
If you want to script to be executed, then you can use the @html.raw method to print out the unencoded string as shown below. This code results in an alert box popping up.
1 2 3 | <span>@Html.Raw(encodedMessage)</span> |
Summary
We looked at the Razor Syntax in this Tutorial. The Razor codes start with @ and you can either use a Razor expressions or Razor code blocks to insert C# code inside HTML Markup. This gives the programmers the ability to create a dynamic web page using C# features like conditional statements, loops etc.