The object is JavaScript’s most fundamental data type and complex data structure. Understanding them is crucial in learning and programming in JavaScript, and Hence in this tutorial, we will explore what a JavaScript object is. We will also learn how to create and use them.
Table of Contents
What is an object in Javascript?
An object is a collection of key-value pairs. Each key-value pair is known as a property. The key is the property’s name, and the value is its value.
Objects provide a way to group several values into a single value. You can group values of other types like strings, numbers, booleans, dates, arrays, etc. An object can also contain other objects. We can quickly build a more complex structure using them.
Object Property
Each key-value pair in the key-value collection is a property of the object.
The Javascript objects can have any number of properties. You can also create an empty object without any properties.
The key or name of the property must be unique. The property name is string (But it can also be a symbol type). Each property must have a unique name.
We store or retrieve the value of an object using its property name (key). Value can be any valid Javascript value. For example, string, number, date, function, or another object.
Types of Properties
There are two kinds of properties in JavaScript. Data Properties & Accessor Properties.
The Data Property maps to a value. The value can be a primitive value, object, or function.
The Accessor property does not map to a value but to one or two accessor functions. The accessor functions (known as getter & setter functions) contain the logic to store or retrieve the value.
How to Create Objects in Javascript
There are several different ways to create new objects in Javascript
- Using Object Literal
- Using a
new
keyword with a constructor function Object.create
method- Using ES6 Class Statement
Object.assign()
method
Creating a Data Property
The following examples use the object literal syntax to create an object in JavaScript.
An object literal is a list of key-value pairs, each separated by a comma and wrapped inside curly braces. Using it is the easiest way to create an object.
1 2 3 4 5 6 7 | var person = { firstName: "Allie", lastName: "Grater", age: 50 }; |
In the example above firstName
, lastName
, & age
are property names and Allie
, Grater
, & 50
are values.
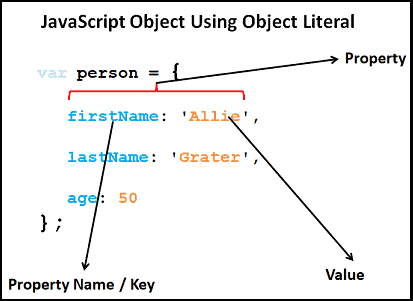
You can refer to the tutorial object literal.
Creating a Accessor Property
The following example shows how to create an object with an Accessor Property color. We do not map color to a value but to get & set JavaScript functions.
The color behaves like a Data Property, but behind the scenes, JavaScript executes the get method when we access the property and the set method when we assign a value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | var car = { //Regular Property //Also known as backing Property to color getter & setter property _color: "blue", // Accessor Property with the name color get color() { //getter return this._color; }, set color(value) { //setter this._color=value; } }; |
Refer to Getters & Setters in JavaScript for more on accessor properties
Object Methods
Methods are like verbs. They perform actions.
Unlike the programming languages like C# & Java, Javascript allows us to store the function in a variable and use it later.
When we assign a function to a property, we call that property a method.
In the following example, we assign a function to the Property fullName
1 2 3 4 5 6 7 8 9 10 | let person = { firstName: "Allie", lastName: "Grater", age: 50, fullName: function() { return this.firstName + " " + this.lastName; } }; |
Property Names
We can use any string as Property Name.
But if the Property name does not follow the JavaScript identifier rules, then we will have to use the square brackets( [ ]
) while declaring them or accessing them. We can also use the quotes while accessing the properties using the object literal syntax
1 2 3 4 5 6 7 8 9 10 | var person = { "full name":"Allie Grater", }; person["date of birth"]="01/01/2010" console.log(person) //{full name: "Allie Grater", date of birth: "01/01/2010"} |
The ES6 allows us to use an expression inside the brackets. Refer to the Computed Property name article to learn more about it.
Accessing the Property
You can access the Property of a JavaScript Object in two ways.
- Dot notation
- Bracket notation
1 2 3 4 | expression.property // dot notation expression[property] // bracket notation |
In dot notation, we use the object’s name followed by a dot and the property’s name. In the following example, we access the firstName and lastName properties using the dot notation.
1 2 3 4 5 6 7 8 9 10 | let person = { firstName: "Allie", lastName: "Grater", }; //Accessing the firstName & lastName Property using the dot notation person.firstName person.lastName |
The dot notation works if the property names follow the JavaScript rules for identifiers. We will have to use the [] bracket notation if they don’t.
In the example below, the full name contains a space, which is not a valid JavaScript identifier. To access it, we need to use the [] bracket notation
1 2 3 4 5 6 7 8 9 | var person = { "full name":"Allie Grater", age:50 }; person["full name"] //Allie Grater person["age"] //50 |
1 2 3 4 5 6 7 8 9 | let obj = { 0: "test" // same as "0": "test" }; // both alerts access the same property (the number 0 is converted to string "0") alert( obj["0"] ); // test alert( obj[0] ); // test (same property) |
Adding or deleting Properties
JavaScript allows us to add or remove Properties to an existing object at any time.
The following code creates a new object person. Later it will add a fullName property, which is a function.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | let person = { firstName: "Allie", lastName: "Grater", age: 50 }; console.log(person); //Adding a new property person.fullName = function() { return this.firstName + " " + this.lastName; }; console.log(person.fullName()); console.log(person); |
Similarly, you can remove a property using the delete statement. In the following example, we delete the age property of the person object.
1 2 3 4 5 6 7 8 9 10 11 12 13 | let person = { firstName: "Allie", lastName: "Grater", age: 50 }; console.log(person); //Deleting age delete person.age; console.log(person); |
Everything is an Object in JavaScript
Javascript has seven primitive data types string
, number
, bigint
, boolean
, undefined
, symbol
, and null
.
Everything else is an object. For example Dates, Functions and arrays are all Objects.
References
Read More
good one