In this tutorial, let us learn how to build a simple hello world example using typescript. We will show you how to compile it using typescript standalone compiler (tsc) and run it using Node. Before getting started with the hello world application, you must install Typescript compiler, NodeJs and Visual Studio Code. We covered this in the previous tutorial on how to Install Typescript. Also, read Introduction to TypeScript
Table of Contents
Typescript Hello World Example
Open the command prompt and create a folder GettingStarted
and then cd into it.
1 2 3 4 | md GettingStarted cd GettingStarted |
Now, Open Visual Studio Code or any text editor. Go to the GettingStarted
folder.
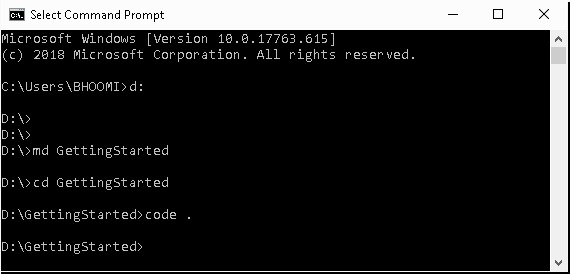
You can open the Visual Studio Code just by typing the following command in the GettingStarted folder
code .
Copy the following code and save the file as helloWorld1.ts
1 2 3 4 | let message:string = "Hello World" console.log(message) |
The message
is a variable of type string
. A variable is a storage for the data. “message”
is the name of the variable. In the code above, it stores the value “Hello World”, which is a string
We use let
(or var
) to create variables in typescript. You will learn more about the variable declaration in our next tutorial
console.log()
writes the whatever the message passed onto it to the console
Compile/Execute TypeScript Programs
Now, go back to the command prompt or click on View -> Terminal in Visual Studio Code menu, which will open the PowerShell terminal window
Typescript codes are compiled using the Typescript compiler ie. tsc. Run the following command to compile
1 2 3 | tsc helloWorld1 |
The tsc
will look for the helloWorld1.ts file and compiles and generates the helloWorld1.js file. Open the file and inspect its content
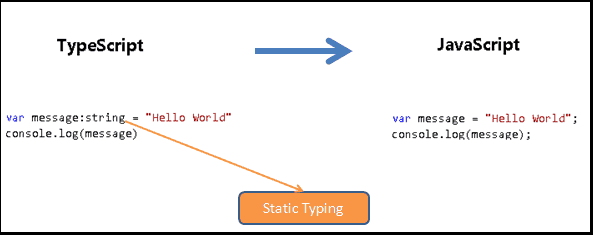
Note that, code is almost the same except for the Type definition “string”, which is removed from the javascript file.
Running the Hello World using nodeJs
We will use the node.js to execute it. Run the following in the command window
1 2 3 | node helloWorld1 |
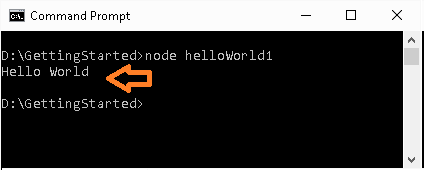
Running TypeScript in the web app
Create the new typescript file hellowWorld2.ts
1 2 3 4 | let message:string = "Hello World" alert(message) |
The only change we have from the above code is we removed console.log()
to alert()
,
The alert()
method displays the message passed onto it in a window, with an ok button. This method works only in the browser, hence we did not use in the previous code.
Compile the helloWorld2 as shown below
1 2 3 | Tsc helloWorld2 |
The above will generate the helloWorld2.js file. Now you can refer this in your HTML file. Create the index.html with the script
tag pointing to the hellowWorld2.js
1 2 3 4 5 6 7 8 9 10 | <!DOCTYPE html> <html> <head> <title></title> <script src="helloWorld2.js"></script> <body></body> </head> </html> |
Open the file using the chrome or any browser, you should be able to see the alert window.
Running in Typescript Playground
You can also run typescript in using the TypeScript Playground. Head over to Typescript Playground
As you type the code, you will see the javascript code is automatically generated in the right-hand side pane.
Open the Developer Console (ctrl-shit-I in chrome) and click on run and see the Hello World appearing in the console window.
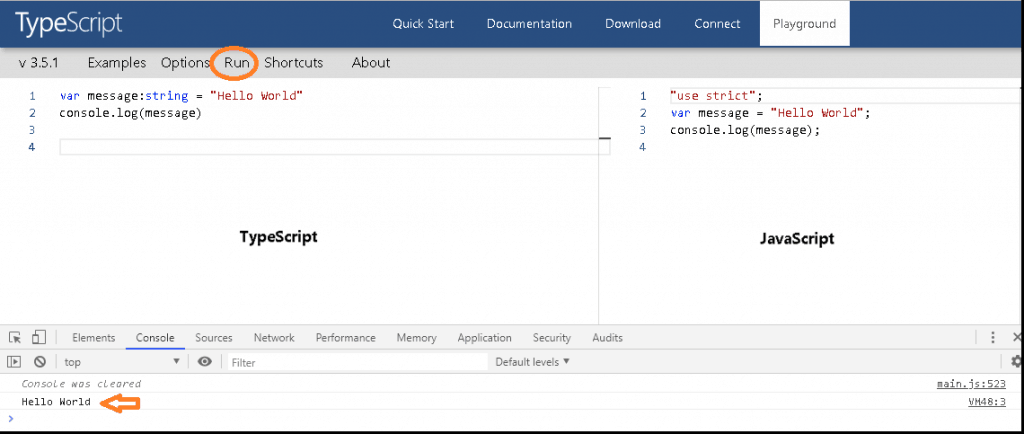
Compile Error
As we mentioned in the previous article on, the typescript compiler warns us if there are any type mismatches.
For Example, create helloWorld3.ts and add the following code. Here we are assigning a string to a number variable.
1 2 3 4 | let message:number = "Hello World" console.log(message) |
Instantly the Visual Studio Code underlines the message in red, indicating the error in the code. Also, when you compile, the compiler will throw the following error.
1 2 3 | tsc helloWorld3.ts |
1 2 3 4 | helloWorld3.ts:1:5 - error TS2322: Type '"Hello World"' is not assignable to type 'number'. 1 var message:number = "Hello World" |
Typescript will generate the javascript code even if there is a compile error. The helloWorld3.ts shown above contains an error but the helloWorld3.js is still produced. This is intentional. This allows us to export our javascript code to typescript and progressively update it to Typescript.
Summary
Now, we have learned how to create a simple Typescript file. Compile it with the Typescript compiler and run it using the Node & Also using the browser