In this guide let us learn the interpolation in Angular with examples. We use interpolation to bind a component property, method or to a template reference variable to a string literal in the view. We also call this as string interpolation. It is one way from component to view as the data flow from the component to view.
Table of Contents
What is Interpolation in Angular
Interpolation allows us to include expressions as part of any string literal, which we use in our HTML. The angular evaluates the expressions into a string and replaces it in the original string and updates the view. You can use interpolation wherever you use a string literal in the view.
Angular interpolation is also known by the name string interpolation. Because you incorporate expressions inside another string.
Interpolation syntax
The Angular uses the {{ }}
(double curly braces) in the template to denote the interpolation. The syntax is as shown below
1 2 3 | {{ templateExpression }} |
The content inside the double braces is called Template Expression
The Angular first evaluates the Template Expression
and converts it into a string. Then it replaces Template expression
with the result in the original string in the HTML. Whenever the template expression changes, the Angular updates the original string again
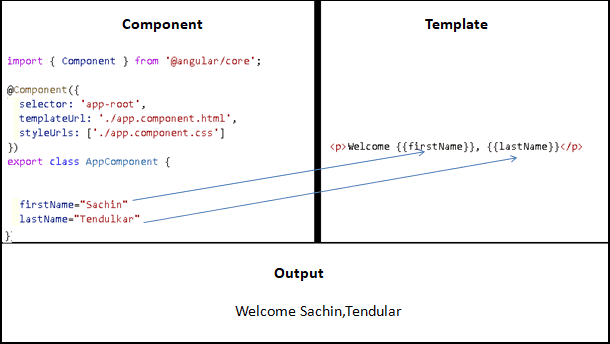
Interpolation Example
Create a new angular application using the following command
1 2 3 | ng new interpolation |
Open the app.component.html
and just add the following code
1 2 3 | {{title}} |
Open the app.component.ts
and add the following code
1 2 3 4 5 6 7 8 9 10 11 12 | import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular Interpolation Example'; } |
Run the app. You will see the “Angular Interpolation Example”
on the screen
In the example above the title
is the Template Expression. We also have title
property in the component. The Angular evaluates {{title}}
and replaces it with the value of the title property from the component class.
If the user changes the title
in the component class, the Angular updates the view accordingly.
The interpolation is much more powerful than just getting the property of the component. You can use it to invoke any method on the component class or to do some mathematical operations etc.
Notes on Interpolation
Interpolation is one-way binding
Interpolation is one way as values go from the component to the template. When the component values change, the Angular updates the view. But if the values changes in the view components are not updated.
Should not change the state of the app
The Template expression should not change the state of the application. The Angular uses it to read the values from the component and populate the view. If the Template expression changes the component values, then the rendered view would be inconsistent with the model
It means that you cannot make use of the following
- Assignments
(=, +=, -=, …)
- Keywords like
new
,typeof
,instanceof
, etc - Chaining expressions with
;
or,
- The increment and decrement operators
++
and--
- bitwise operators such as
|
and&
The expression must result in a string
Interpolation expression must result in a string. If we return an object it will not work. If you want to bind the expression that is other than a string (for example – boolean), then Property Binding is the best option.
Works only on Properties & not attributes
Interpolation and property binding can set only properties, not attributes. For Attributes use attribute binding
Examples of interpolation
You can use interpolation to invoke a method in the component, Concatenate two string, perform some mathematical operations or change the property of the DOM element like color, etc.
Invoke a method in the component
We can invoke the component’s methods using interpolation.
1 2 3 4 5 6 7 8 9 10 11 12 | //Template {{getTitle()}} //Component title = 'Angular Interpolation Example'; getTitle(): string { return this.title; } |
Concatenate two string
1 2 3 4 5 6 | <p>Welcome to {{title}}</p> <p>{{ 'Hello & Welcome to '+ ' Angular Interpolation '}}</p> <p>Welcome {{firstName}}, {{lastName}}</p> <p>Welcome {{getFirstName()}}, {{getLastName()}}</p> |
Perform some mathematical operations
1 2 3 4 5 6 7 8 9 10 11 | <h2>Mathematical Operations</h2> <p>100x80 = {{100*80}}</p> <p>Largest: {{max(100, 200)}}</p> //Component max(first: number, second: number): number { return Math.max(first, second); } |
Bind to an element property
We can use it to bind to a property of the HTML element, a component, or a directive. in the following code, we bind to the style.color
property of the <p>
element. We can bind to any property that accepts a string.
1 2 3 4 | <p>Show me <span class = "{{giveMeRed}}">red</span></p> <p style.color={{giveMeRed}}>This is red</p> |
Bind to an image source
1 2 3 | <div><img src="{{itemImageUrl}}"></div> |
href
1 2 3 | <a href="/product/{{productID}}">{{productName}}</a> |
Use a template reference variable
You can also use the template reference variable. The following example creates a template variable #name
to an input box. You can use it get the value of the input field {{name.value}}
1 2 3 4 5 | <label>Enter Your Name</label> <input (keyup)="0" #name> <p>Welcome {{name.value}} </p> |
We also use (keyup)="0"
on the input
element. It does nothing but it forces the angular run the change detection, which in turn updates the view.
The Angular updates the view, when it runs the change detection. The change detection runs only in response to asynchronous events, such as the arrival of HTTP responses, raising of events, etc. In the example above whenever you type on the input box, it raises the keyup
event. It forces the angular run the change detection, hence the view gets the latest values.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | //Template <p>items</p> <ul> <li *ngFor="let d of items"> {{ d.name }} </li> </ul> //Component items= [ new item(1, 'Mobile'), new item(2, 'Laptop'), new item(3, 'Desktop'), new item(4, 'Printer') ] class item { code:string name:string constructor(code,name) { this.code=code; this.name=name } } |
Cross-site Scripting or XSS
Angular Sanitizes everything before inserting into DOM, thus preventing Cross-Site Scripting Security bugs (XSS). For example values from the component property, attribute, style, class binding, or interpolation, etc are sanitized. The script
in the following example is not invoked but shown as it is.
1 2 3 4 5 6 7 8 9 | //Template <p>{{script}}</p> <p>{{div}}</p> //Component script ='<script>alert("You are hacked")</script>' div='<div>this is a div</div>'; |
NgNonBindable
Use ngNonBindable
to tell Angular not to compile or bind the contents of the current DOM element. I.e any expression is not evaluated but shown as it is.
1 2 3 4 5 6 7 8 | <p>Evaluate: {{variable}}</p> <p ngNonBindable>Do not evaluate: {{variable}}</p> <p>Angular uses {{ variable }} syntax for Interpolation</p> <p ngNonBindable>Angular uses {{ variable }} syntax for Interpolation</p> |
Use Pipes
You can make use of Angular Pipes to transform the expression result. Like converting to an uppercase, date formats, adding currency symbols, etc
1 2 3 4 5 | <p>uppercase pipe: {{title | uppercase}}</p> <p>pipe chain: {{title | uppercase | lowercase}}</p> <p>json pipe: {{items | json}}</p> |
You can make use of a safe navigation operator ( ? ) to guards against null
and undefined
values.
The following code results in an error because there is no nullItem
1 2 3 4 5 | <p>The item name is: {{nullItem.Name}}</p> TypeError: Cannot read property 'itemName' of undefined |
Use a safe navigation operator and the error goes away. Angular replace it with an empty string
1 2 3 | <p>The null item name is {{nullItem?.itemName}}</p> |
The non-null assertion operator
Typescript enforces the strict null checking if you enable the --strictNullChecks
flag in your tsconfig.json
. Under strict null check any variable not defined or null results in a compiler error. The type checker also throws an error if it can’t determine whether a variable will be null or undefined at runtime
You can use the non-null assertion operator to inform typescript not to throw any compile errors. Note that it is a compile-time feature & not runtime.
1 2 3 | The item's name is: {{item!.name}} |
References
- Angular Tutorial
- Angular Data Binding
- Template Syntax
- Property Binding
- Event Binding
- Two-way binding
- Template Reference Variable
- Angular Pipes
- Cross-Site Scripting
- String Interpolation in Typescript
Summary
Interpolation in Angular is a simple, yet powerful feature. It allows us to embed expressions in the string literal, Hence we can dynamically generate the string literal using the values from the component class.
thanks
Hi there, thanks for the content, I really enjoy it and make a great use of it. But there is a small mistake in “The safe navigation operator ( ? )” section. The first code example shouldnt contain ‘?’ operator and the second code example should contain ‘?’ operator, whereas the exact opposite happened :).
Fyi
Its tsconfig.json file not sconfig.json file in “The non-null assertion operator”.
Thanks. Updated the code