Arrays in JavaScript allow us to group several values in a single variable. Take the example of the scores obtained by a bunch of students in a subject. We can create a variable for each student to store the score, but that is cumbersome to handle and maintain. The arrays allow us to create a single variable and keep all its scores. You can think of an array as a bag containing multiple items.
Table of Contents
- What is a JavaScript Array
- Benefits of Using Javascript Arrays
- Creating Arrays in JavaScript
- Finding the length of an array
- Reading an item from Array.
- Modifying Array Elements
- Adding and Removing Elements from Array
- Looping through Array Elements
- Arrays are objects
- Check if a variable is an array.
- Summary
- Reference
What is a JavaScript Array
JavaScript array is a data structure that can store ordered collection items of various data types. An ordered collection of items means that the items follow a specific order. The order is independent of the value of the item. To maintain order, each item is given a nonnegative integer starting with 0, known as the index of the array. We use the index to access the array element.
For Example, you can compare a JavaScript array to a row of boxes, each of which can hold a single element. Every box is given a number starting with 0. This number is what we call the Index of the Array. The index number is what we use to access a particular value from the array.
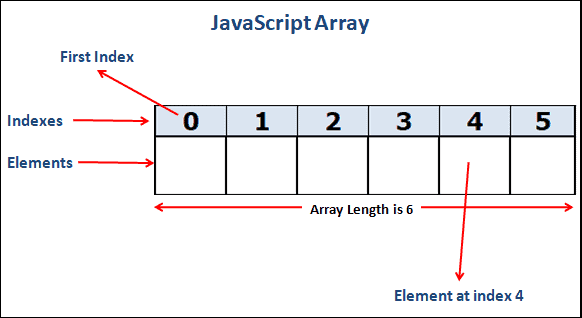
We can store anything in the JavaScript Array. Numbers, strings, boolean, or objects. We can even store another array in an array.
Benefits of Using Javascript Arrays
There are many benefits to using arrays. Arrays are a great way to organize and manipulate related data.
- Flexibility: JavaScript Arrays are very flexible. We can use them to store different data types, such as numbers, strings, objects, etc.
- Efficiency: Arrays can store, retrieve, and manipulate data quickly and efficiently.
- Searching: Arrays are great for searching data, as you can quickly search for items in an array using the element’s index.
- Sorting: Arrays can be easily sorted quickly, making them ideal for sorting data.
- Manipulation: Arrays are great for manipulating data, as they can add, remove, and modify elements quickly and easily.
Creating Arrays in JavaScript
The simplest way to create an array is to use the array literal notation.
The syntax is as follows.
1 2 3 | let arrayName = [value1, value2, value3, ...]; |
Where arrayName is the name of the array.
Value1, value2, value3, etc., are the values we want to store in the array. The values must be enclosed in square brackets [ ]. You can store any number of values. A comma must separate each value.
The following code creates an array with five values: A number, string, Boolean, null, and an object.
1 2 3 | const myArray = [1, "Hello" , true , null , {id:"1", name:"Bill"} ]; |
The following image shows how JavaScript stores the above values in the array.
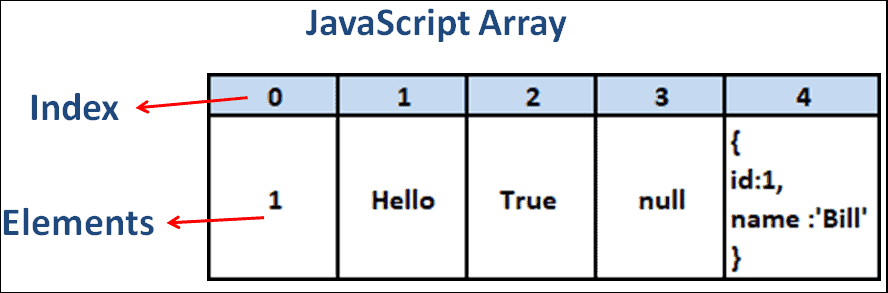
Finding the length of an array
The length property of the array object returns the number of elements in the array. But if the array is sparse, then the length also includes the empty slots ( refer to dense and sparse arrays ).
1 2 3 4 5 | const books = ["Ulysses", "Don Quixote", "War and Peace", "Moby Dick"]; console.log(books.length) //4 |
We can also use the length property to increase or shorten the array’s length. The code below increases the length to ten from four.
1 2 3 4 5 6 | //Example const books = ["Ulysses", "Don Quixote", "War and Peace", "Moby Dick"]; books.length = 10 console.log(books.length) //10 |
You can decrease the array’s length by setting it to less than the number of elements in the array. This will remove all the elements beyond the length.
The code below decreases the length to two from four. The JavaScript truncates the array and removes the last two elements.
1 2 3 4 5 6 7 | //Example const books = ["Ulysses", "Don Quixote", "War and Peace", "Moby Dick"]; books.length = 2 console.log(books.length) //2 console.log(books) //["Ulysses", "Don Quixote"] |
Hence, by setting the length to zero, you can empty the array
1 2 3 4 5 6 | const books = ["Ulysses", "Don Quixote", "War and Peace", "Moby Dick"]; books.length = 0 console.log(books.length) //0 console.log(books) //[""] |
You can read more about the length of an array in JavaScript.
Reading an item from Array.
We can access any item in a JavaScript array using its index number. The syntax uses the square bracket with the element’s Index number inside it.
For Example, myArray[2]
returns the element at index two from the array myArray
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | const myArray = [1, "Hello" , true , null , {id:"1", name:"Bill"} ]; //first element at index 0 myArray[0] //1 //second element at index 1 myArray[1] //Hello myArray[2] //True myArray[3] //Null //fifth element at index 4 myArray[4] //{id:"1", name:"Bill"} |
Accessing the First Array Element
1 2 3 | myArray[0] |
Accessing the Last Element
1 2 3 | myArray[myArray.length] |
At method
We can also use the at
method to read the item from the array. The at method is a recent addition to JavaScript. Hence, some browsers may not support it.
array.at(i)
returns the element from the index position i
. It is similar to array[I]
.
You can invoke at
method with a negative number will read the array from the end. For example, array.at(-i)
read the ith
element from the end of the array. i.e., it will read array[array.length-i]
element.
1 2 3 4 5 6 7 8 9 10 | const myArray = [1, "Hello" , true , null , {id:"1", name:"Bill"} ]; myArray.at(0) //1 same as myArray[0] myArray.at(1) //Hello same as myArray[1] //Reading from the end of the array myArray.at(-1) // {id:"1", name:"Bill"} same as myArray[myArray.length-1] myArray.at(-2) // null same as myArray[myArray.length-2] |
Modifying Array Elements
You can modify an element at any index by assigning a value.
1 2 3 4 5 6 | const books = ["Ulysses", "Don Quixote", "War and Peace", "Moby Dick"]; books.length //4 books[0] ="Invisible Man" //Modifying an element |
You can also add an element to the new index. The array below has elements starting at index 0 to 3 (A total of four elements.) We assign a value at index 4, which does not exist. The JavaScript adds a new element at the location and increases the array length to 5.
1 2 3 4 5 6 7 8 | const books = ["Ulysses", "Don Quixote", "War and Peace", "Moby Dick"]; books.length //4 books[4] ="Invisible Man" //Adding a new element at Index 4 books.length // 5 . Now there are five elements in the array |
The Books array below has only four elements. But we assign a value at index 40. This is valid, and the value is inserted at index 40. Thus, the length of the array becomes 41. The index elements b/w 4 to 39 contain no element. Such an array is called a sparse array.
1 2 3 4 5 6 7 | const books = ["Ulysses", "Don Quixote", "War and Peace", "Moby Dick"]; books.length //4 books[40] ="Invisible Man" //Adding a new element at Index 40 books.length // 41 . Now there are 41 elements in the array. |
Adding and Removing Elements from Array
Once you have created a JavaScript array, you can add and remove elements from it
Adding a new element at the end of an array
To add an element to an array, you can use the Array.push()
method. This method allows you to add elements to the end of an array.
For example, if you wanted to add the number 6 to the end of the numbers
array, you could use the following syntax:
1 2 3 4 5 6 | let numbers = [1, 2, 3, 4, 5]; numbers.push(6); console.log(numbers); // [1,2,3,4,5,6] |
You can push multiple items to the end of the array. The code below pushes values 6, 7, and 8 to the numbers
array
1 2 3 4 5 6 | let numbers = [1, 2, 3, 4, 5]; numbers.push(6,7,8); console.log(numbers); // [1,2,3,4,5,6,7,8] |
Add a new element at the start of the array
The Array.unshift
method allows you to add elements to the start of an array.
The following example adds the number 6 to the start of the numbers
array.
1 2 3 4 5 6 | let numbers = [1, 2, 3, 4, 5]; numbers.unshift(6); console.log(numbers); // [6,1,2,3,4,5] |
You can insert multiple items at the start of the array. The code below inserts values 6, 7, and 8 into the numbers
array at the start
1 2 3 4 5 6 | let numbers = [1, 2, 3, 4, 5]; numbers.unshift(6,7,8); console.log(numbers); // [6,7,8,1,2,3,4,5] |
Adding an element at a particular index
The splice
method changes an array’s contents by removing or replacing existing elements and adding new ones.
The following code shows the syntax of the splice method.
1 2 3 | splice(start, deleteCount, item1, item2, itemN) |
The splice
method usually accepts three arguments when adding an element:
- The start is the index of the array where we start changing the array.
- deleteCount is the number of items to be removed from the start index
- item1, item2, itemN, etc., are the elements to add to the array at the index start
The code below inserts the value 4
at index 3
. It will remove 0
any elements from the array.
1 2 3 4 5 6 | let numbers = [1, 2, 3, 5, 6 ]; numbers.splice(3,0,4); // Start 3 Number of elements to delete= 0 items to insert 4 console.log(numbers); // [1,2,3,4,5,6] |
The following code inserts 9,10 & 11 at index location 3. It also removes the three elements starting from location 3.
1 2 3 4 5 6 | let numbers = [1, 2, 3, 5, 6 ]; numbers.splice(3 ,3, 9,10,11); //start=3 Number of elements to delete= 3 items to insert 9, 10, 11 console.log(numbers); // [ 1, 2, 3, 9, 10, 11 ] |
The code below inserts 9,10,11 at index 3. It will not remove any items
1 2 3 4 5 6 | let numbers = [1, 2, 3, 5, 6 ]; numbers.splice(3,0,9,10,11); ////start=3 Number of elements to delete= 0 items to insert 9, 10, 11 console.log(numbers); // [ 1, 2, 3, 9, 10, 11, 5, 6 ] |
Remove an element from the end of the Array
We use the pop() method to remove an element from the end of the array. It returns the value it just removed
The code below removes the last element, 6, from the array.
1 2 3 4 5 6 7 8 | let numbers = [1, 2, 3, 4, 5, 6]; numbers.pop(); // 6 removed console.log(numbers) //[ 1, 2, 3, 4, 5 ] numbers.pop(); // 5 removed console.log(numbers) //[ 1, 2, 3, 4 ] |
The following code below creates an array of numbers from zero to six. It then sets the value ten at index location ten. The array length will become 11 with no values at locations 7, 8, & 9.
1 2 3 4 5 6 7 8 9 10 11 | let numbers = [0,1, 2, 3, 4, 5, 6]; numbers[10]=10 //10 added at index 10. The index locations 7,8,&9 are empty console.log(numbers) //[ 0, 1, 2, 3, 4, 5, 6, <3 empty items>, 10 ] numbers.pop(); // 10 removed console.log(numbers) //[ 0, 1, 2, 3, 4, 5, 6, <3 empty items>] numbers.pop(); // 9the element removed which is empty console.log(numbers) //[ 0, 1, 2, 3, 4, 5, 6, <2 empty items>] |
Remove an element from the start of the Array.
We use the shift() method to remove an element from the beginning of the array. It returns the value it just removed.
The code below removes the first element, 0, from the array.
1 2 3 4 5 6 7 8 | let numbers = [0, 1, 2, 3, 4, 5]; numbers.shift(); // 0 removed console.log(numbers) //[ 1, 2, 3, 4, 5 ] numbers.shift(); // 1 removed console.log(numbers) //[ 2, 3, 4, 5 ] |
Removing an element from a particular index
The splice method can also remove an item from the array. So that you know, all you need to do is to pass only the first two arguments. i.e., start index and no of elements to remove.
The code below removes the two items from the array starting from index 2.
1 2 3 4 5 | let numbers = [0, 1, 2, 3, 4, 5]; numbers.splice(2,2); // it will remove two items starting from the inde 2 ( 2 & 3) console.log(numbers) //[ 0, 1, 4, 5 ] |
Removes the first item similar to the shift
1 2 3 4 5 | let numbers = [0, 1, 2, 3, 4, 5]; numbers.splice(0,1); // removes the first item. Similar to Shift console.log(numbers) //[ 1, 2, 3, 4, 5 ] |
Removes the last item similar to pop
1 2 3 4 5 | let numbers = [0, 1, 2, 3, 4, 5]; numbers.splice(numbers.length-1,1); // removes the last item. Similar to pop console.log(numbers) //[ 1, 2, 3, 4 ] |
Looping through Array Elements
Another important array task is to loop through all elements of the array. You can achieve that in two ways. They are
- For Loop
- For of
For loop
The For loop is the simplest way to iterate over the elements of the array.
1 2 3 4 5 6 | let numbers = [0,1,2,3,4,5]; for (let i = 0; i < numbers.length; i++) { console.log(numbers[i]) // 0 1 2 3 4 5 } |
For off loop
The for of
statement loops through the values of an iterable object
1 2 3 4 5 6 | let numbers = [0,1,2,3,4,5]; for (let num of numbers) { console.log(num) // 0 1 2 3 4 5 } |
Arrays are objects
Arrays are a special type of object in JavaScript.
There is no explicit array data type for arrays in JavaScript. The array we use is an object extended to work with arrays. The array object has methods to manipulate arrays. It has methods to sort, filter, reverse, etc.
A JavaScript object is a collection of key-value pairs. Each key-value pair in the key-value collection is a property of the object. In the case of an array, each element is a property with indexes as their names (key). We access an object property using the bracket notation obj[key]. The array element access uses the same syntax, with the index as the key.
The typeof operator returns “object” for the arrays.
1 2 3 4 5 | let numbers = [0,1,2,3,4,5] console.log(typeof(numbers)) //object |
Because they are objects, we can add properties & methods to them. The code below adds the printMe function to the numbers array.
1 2 3 4 5 6 7 8 9 | let numbers = [0,1,2,3,4,5] numbers.printMe= function() { for (let i = 0; i < this.length; i++) { console.log(this[i]) } } |
JavaScript for in
loops enumerable properties of an object. Since arrays are objects, we can use them to loop through their properties.
The code below is used for in
to loop through the keys of an array. Notice that it has a printed printMe
function along with the array items.
1 2 3 4 5 6 7 8 9 10 11 12 13 | let numbers = [0,1,2,3,4,5] numbers.printMe= function() { for (let i = 0; i < this.length; i++) { console.log(this[i]) } } for (let key in numbers) { console.log( numbers[key] ); // 0 1 2 3 4 5 } |
If we supply a non-integer value to the array operator, it will not create an array element. But it will create a property in the object representing the array.
1 2 3 4 5 6 7 8 9 10 | const books = ["Ulysses", "Don Quixote"]; books[2.5] = 'War and Peace'; console.log(books.length); // 2 & not ; console.log(books.hasOwnProperty('2.5')); // true console.log(books) //[ 'Ulysses', 'Don Quixote', '2.5': 'War and Peace' ] |
Check if a variable is an array.
You can tell whether a given variable is an array or not using the isArray or the instanceof operator. We cannot use the Typeof operator to check for an array because it returns an object.
The syntax of isArray
and instanceof
is as shown below.
1 2 3 4 5 | Array.isArray(obj) obj instanceof Array |
Examples
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | number=[1,2,3,4] a= {} b=[] function isArray(obj) { if (obj) return Array.isArray(obj) return false } console.log(isArray(number)) //true console.log(isArray("number")) //false console.log(isArray(a)) //fasle console.log(isArray(b)) //true |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | number=[1,2,3,4] a= {} b=[] function isArray(obj) { if (obj) return obj instanceof Array return false } console.log(isArray(number)) //true console.log(isArray("number")) //false console.log(isArray(a)) //fasle console.log(isArray(b)) //true |
Summary
JavaScript array is a data structure that can store ordered collection items of various data types
The simplest way to create an array is to use the array literal notation. For Example let arrayName = [value1, value2, ...];
Array.length return the length of the array. The count might not be the same as the number of elements in the array, but it includes the empty elements (sparse arrays).
We can read/modify any item in an array using its index number.
push
method pushes new element at the end of the array
shift
inserts new element at beginning of the array
pop
method removes the element from the end of the array
unshift
removes an element from the beginning of the array
Splice
method modifies or removes elements from any location
Use for loop or for of loop to traverse all array elements. Avoid for in loop
You can tell if a given variable is an array or not using the isArray
or using the instanceof
operator.
There is no explicit array data type for arrays in JavaScript. The array we use is an object extended to work with arrays.