In this article, we will look at the role of program.cs in ASP.NET Core. It is the entry point of our app, where we configure the host. We also configure and register required services and configure middleware pipelines & endpoints.
The program class has changed in .NET 6.0. Visit program class in older versions of ASP.NET Core to read about the older version. You can also refer to the startup class.
Table of Contents
What is Program.cs ?
The Program
class is the entry point for the ASP.NET Core application. It contains the application startup code.
It is where we
- Configure and register the services required by the app
- Register middleware components and configure the app’s request-handling pipeline.
What is a host?
On startup, ASP.NET Core apps configure and launch a host
.
You can think of a host
as a wrapper around your application. It is responsible for the startup and lifetime management of the application. The host contains the application configuration and the Kestrel server (an HTTP server) that listens for requests and sends responses. It also sets up the logging, dependency injection, configuration, request processing pipeline, etc.
There are three different hosts capable of running an ASP.NET Core app.
- WebApplication (or Minimal Host)
- Generic Host
- WebHost
WebHost was used in the initial versions of ASP.NET Core. Generic Host replaced it in ASP.NET Core 3.0. WebApplication was introduced in ASP.NET Core 6.0.
What is WebApplication (Minimal Host)
The WebApplication
is the core of your ASP.NET Core application. It contains the application configuration and the HTTP server (Kestrel) that listens for requests and sends responses.
WebApplication behaves similarly to the Generic Host, exposing many of the same interfaces but requiring fewer configure callbacks.
We need to configure the WebApplication before we run it. There are two essential tasks that we need to perform before running the app.
- Configure and add the services for dependency injection.
- Configure the request pipeline, which handles all requests made to the application.
We do all these things in the program.cs
Understanding program.cs
Create a new ASP.NET core application using the ASP.NET Core Empty template. Open the program.cs. You will see the following lines.
1 2 3 4 5 6 7 8 | var builder = WebApplication.CreateBuilder(args); var app = builder.Build(); app.MapGet("/", () => "Hello World!"); app.Run(); |
These four lines contain all the initialization code you need to create a web server and start listening for requests.
The first thing you notice in this file is that no main method exists. The main method is the entry point of every C# program. But C#9 introduced the top-level statements feature, where you do not have to specify a main method. This feature allows us to create one top-level file containing only statements. It will not have to declare the main method or namespace. That top-level file will become the entry point of our program.
The program file creates the web application in three stages. Create, Build, and Run
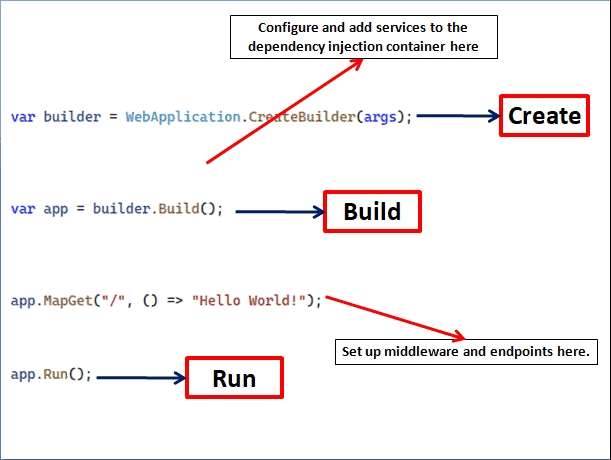
The earlier versions of ASP.NET Core created two files. One is program.cs, and the other is startup.cs (known as startup class). The program.cs is where we configured the host, and the startup class is where we used to configure the application. Since ASP.NET core 6, both processes are simplified and merged into a program.cs.
Create
The first line of the code creates an instance of the WebApplication
builder.
1 2 3 | var builder = WebApplication.CreateBuilder(args); |
The CreateBuilder is a static method of WebApplication class. It sets up a few basic features of the ASP.NET platform by default, including
- Sets up HTTP server (Kestrel)
- Logging
- Configuration
- Dependency injection container
- Adds the following framework-provided services
This method returns a new instance of the WebApplicationBuilder class.
We use the WebApplicationBuilder
object to configure & register additional services.
WebApplicationBuilder
 exposes the ConfigurationManager
 type, which exposes all the current configuration values. We can also add new configuration sources.
It also exposes a IServiceCollection
. We use this to add services to the DI container.
We then call the build method.
Build
The build method of the WebApplicationBuilder class creates a new instance of the WebApplication class.
We use the instance of the WebApplication
to set up the middleware’s and endpoints.
The template has set up one middleware component using the MapGet extension method.
1 2 3 | app.MapGet("/", () => "Hello World!"); |
Run
The run method of the WebApplication
instance starts the application and listens to http requests.
Program.cs in Various Project Templates
.NET SDK Provides several project templates for creating a new ASP.NET core application. The most commonly used templates are
- ASP.NET Core Empty
- ASP.NET Core Web App (Razor pages)
- ASP.NET Core Web App (Model-View-Controller)
- ASP.NET Core Web API
ASP.NET Core Web App
The following program class is from the template ASP.NET Core Web App (Razor pages).
The code uses the extension method AddRazorPages
to register Razor page-related services. Note that it is added before calling the build method.
After the build method, several middleware components are added.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 | var builder = WebApplication.CreateBuilder(args); //Create // Add services to the container. builder.Services.AddRazorPages(); var app = builder.Build(); //Build // Configure the HTTP request pipeline. if (!app.Environment.IsDevelopment()) { app.UseExceptionHandler("/Error"); // The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts. app.UseHsts(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting(); app.UseAuthorization(); app.MapRazorPages(); app.Run(); //Run |
ASP.NET Core Web App (Model View Controller)
The following program class is from the ASP.NET Core Web App (Model View Controller) template.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | var builder = WebApplication.CreateBuilder(args); // Add services to the container. builder.Services.AddControllersWithViews(); var app = builder.Build(); // Configure the HTTP request pipeline. if (!app.Environment.IsDevelopment()) { app.UseExceptionHandler("/Home/Error"); // The default HSTS value is 30 days. You may want to change this for production scenarios, see https://aka.ms/aspnetcore-hsts. app.UseHsts(); } app.UseHttpsRedirection(); app.UseStaticFiles(); app.UseRouting(); app.UseAuthorization(); app.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); app.Run(); |
ASP.NET Core Web API
The following program class is from the ASP.NET Core Web Api template.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | var builder = WebApplication.CreateBuilder(args); //Create // Add services to the container. builder.Services.AddControllers(); // Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle builder.Services.AddEndpointsApiExplorer(); builder.Services.AddSwaggerGen(); var app = builder.Build(); //Build // Configure the HTTP request pipeline. if (app.Environment.IsDevelopment()) { app.UseSwagger(); app.UseSwaggerUI(); } app.UseHttpsRedirection(); app.UseAuthorization(); app.MapControllers(); app.Run(); //Run |
Summary
The Main
method of the program.cs
class is the entry point of our application. It configures & builds the Web host. The web host is responsible for running our app. Most of the plumbing required to configure host is already done for us in the createdefaultbuilder
method, which is invoked in the Main
method. We can further add our custom configuration is startup class, which we cover in the next tutorial.
Useful Information. Thanks for sharing