There are several ways in which you can merge arrays in Javascript. You can use the spread operator, concat method, or use the well tested for loop and push technique. In this post, let us explore them in detail.
Table of Contents
Merging Arrays in JavaScript
The Array in Javascript is a data structure that can store ordered collection items of various data types.
There are situations where you want to merge two are more arrays into a single array. For Example, you may get arrays from different data sources and need to join them before doing something useful with them.
There are five distinct ways to merge arrays in JavaScript
- Spread Operator
- The
concat()
method - push method
- for each
Merging Arrays using the spread operator
The spread operator is the most preferred and efficient way to merge two arrays in JavaScript. spread operator takes an iterable object like an array, object, string, etc., and spreads its contents.
The spread syntax consists of three dots followed by the name of the array or object (iterable).
1 2 3 | [...numbers] |
For example, let’s say you have an array of numbers called numbers
. To spread it, we will use the statement ...numbers
. Javascript will replace …numbers with the content of the array.
1 2 3 4 5 6 7 | const numbers = [1, 2, 3]; console.log(numbers) //[ 1, 2, 3 ] This is array console.log(...numbers) //1 2 3 This is just a sequence of numbers |
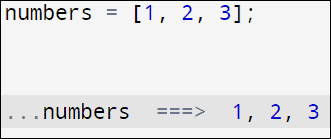
We can merge two or more arrays by using the array literal and spreading out the existing array inside the array literal. The syntax is as shown below.
const mergedArray = […array1, …array2, …array3, …arrayN];
Where array1, array2, array3, etc., are arrays. You can merge any number of arrays.
The code below joins the two arrays into a single array of numbers.
1 2 3 4 5 6 7 8 | const num1Arr=[1,2,3] const num2Arr=[4,5,6] const numbers = [...num1Arr, ...num2Arr]; console.log(numbers) //[ 1, 2, 3, 4, 5, 6 ] |
The spread operator always returns the new array. It will not alter the existing arrays.
Merging Arrays with the Concat Method
The Concat method takes two or more arrays as arguments and returns a new merged array that contains the elements of all the arrays.
Concat method does not modify the original arrays i.e. it does not mutate the original array. It will always return a new array.
The following example shows how to merge two arrays.
1 2 3 4 5 6 7 8 | const num1Arr=[1,2,3] const num2Arr=[4,5,6] const mergedArray = num1Arr.concat(num2Arr); console.log(mergedArray) //[ 1, 2, 3, 4, 5, 6 ] |
You can merge any number of arrays. The code below merges three arrays.
1 2 3 4 5 6 7 8 9 | const num1Arr=[1,2,3] const num2Arr=[4,5,6] const num3Arr=[7,8,9] const mergedArray = num1Arr.concat(num2Arr,num3Arr); console.log(mergedArray) //[ 1, 2, 3, 4, 5, 6, 7, 8, 9 ] |
Calling Array.prototype.concat
directly.
1 2 3 4 5 6 7 8 9 | const num1Arr=[1,2,3] const num2Arr=[4,5,6] const num3Arr=[7,8,9] const mergedArray = Array.prototype.concat(num1Arr,num2Arr,num3Arr); console.log(mergedArray) //[ 1, 2, 3, 4, 5, 6, 7, 8, 9 ] |
Like the spread operator, the contact always returns the new array. It will not alter the existing arrays.
Merging Arrays with the Push Method
The push method is another way to merge two arrays in JavaScript.
The array push method inserts a new elements to the end of an existing array. It takes the element to be added as an argument and returns the array’s new length.
We can insert one or more arrays into another array using push method along with the spread operator.
In the following example, we merge OrigArray with numArr1.
1 2 3 4 5 6 7 8 9 | let OrigArray = [1,2,3]; let numArr1 = [4,5,6]; //Merging numArr1 with OrigArray using combination of push & spread OrigArray.push(...numArr1); console.log(OrigArray) |
The code below merges two arrays with the OrigArray
.
1 2 3 4 5 6 7 8 9 10 | let OrigArray = [1,2,3]; let numArr1 = [4,5,6]; let numArr2 = [7,8,9]; //Merging two arrays with with OrigArray OrigArray.push(...numArr1, ...numArr2); console.log(OrigArray) // [ 1, 2, 3, 4, 5, 6, 7, 8, 9] |
Note that the push method mutates the original array, i.e., it modifies the original array.
Unshift method
Unshift method is very similar to the push method except that it inserts a new value at the start of the array.
But remember unshift method needs to shift all existing elements to a higher index to make room for the new element at the start of the array. This makes it slower compared to all other methods. This is more noticeable as the array gets larger. Push method is faster as it inserts the element to end of the array, hence no need to shift elements.
1 2 3 4 5 6 7 8 9 10 | let OrigArray = [1,2,3]; let numArr1 = [4,5,6]; let numArr2 = [7,8,9]; //Merging two arrays with with OrigArray OrigArray.unshift(...numArr1, ...numArr2); console.log(OrigArray) // [ 4, 5, 6, 7, 8, 9, 1, 2, 3,] |
For Loop
Using For Loop, we can iterate through an array and insert its values into another array or a new array. We can also run some custom checks on the inserted elements. It will help us filter and transform the elements before merging the arrays.
For Loop is very flexible and gives complete control to you. And its performance is as good as the push and spread operator.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | let numArr1 = [1,2,3]; let numArr2 = [4,5,6]; let numArr = mergeArray(numArr1,numArr2) console.log(numArr) //[ 1, 2, 3, 4, 5, 6 ] function mergeArray(first, second) { for(let i=0; i<second.length; i++) { //You can run custom logic here //We are pushing into the existing array. You can also create a new array first.push(second[i]); } return numArr1; } |
ForEach loop
ForEach method is similar and is as flexible as for loop. We can loop through one of the arrays and push its values into an array. Just like For Loop, forEach is also is very flexible and gives complete control to you.
The code below merges numArr1
with numArr
.
1 2 3 4 5 6 7 8 9 10 | let numArr = [1,2,3]; let numArr1 = [4,5,6]; numArr1.forEach((element) => { numArr.push(element) }); console.log(numArr) //[ 1, 2, 3, 4, 5, 6 ] |
Merging two arrays into a new array.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | let numArr1 = [1,2,3]; let numArr2 = [4,5,6]; let mergedArray=[] numArr1.forEach((element) => { mergedArray.push(element) }); numArr2.forEach((element) => { mergedArray.push(element) }); console.log(mergedArray) //[ 1, 2, 3, 4, 5, 6 ] |
Transform and Merge Arrays
There are several use cases where we would like to transform the array before we merge them. For Example, we would like to modify the values of the existing array, filter some of the elements, etc.
JavaScript arrays offer several methods like map, filter, reduce, or sort to transform the array. You can then merge it with another array using the concat method or the spread operator (…).
Array Map method
The Array map method is another alternative to merge arrays when you wish to transform the array before joining. The map method returns a new array after changing the given array with new values based on specific conditions. You can then use the conact method to create a new merged array.
The code below multiplies each array element with two and merges them using the concat.
1 2 3 4 5 6 7 8 9 10 11 | const numArr1 = [1, 2, 3]; const numArr2 = [4, 5, 6]; const mergedArray = numArr1.map(element => element * 2) .concat( numArr2.map(element => element * 2) ); console.log(mergedArray); // Output: [2, 4, 6, 8, 10, 12] |
Array Filter method
The array filter can be used to filter out unwanted values before merging them
1 2 3 4 5 6 7 8 9 10 | const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]; const evenNumbers = numbers.filter(number => number % 2 === 0); mergedArray = [ ...numbers.filter(number => number % 2 === 0), ...numbers.filter(number => number % 2 != 0 ) ] console.log(mergedArray) //[ 2, 4, 6, 8, 10, 1, 3, 5, 7, 9 ] |
You can also use the sort & reduce methods to transform the array
Summary
- In JavaScript, merging arrays involves combining two or more arrays into a single array.
- The spread operator (…), push() method, and concat() method is commonly used to merge arrays.
- You can also use the for loop or for each loop to merge arrays, which offers maximum flexibility.
- You can combine Map, filter, sort, and reduce methods to transform the array before merging.
This is a useful site. I would like to say Thank You.