Typescript Types (or data types) bring static type checking into the dynamic world of Javascript. In this tutorial, we learn what is Typescript types are. We also introduce you to the basic data types that Typescript supports.
Table of Contents
What is a Data Type?
The Type or the Data Type is an attribute of data that tells us what kind of value the data has. Whether it is a number, string, boolean, etc. The type of data defines the operations that we can do on that data.
Take a look at the following example. The code declares two numbers and adds them using the +
operator. The runtime correctly identifies the data type as a number and runs the arithmetic addition on them.
1 2 3 4 5 6 | let num1=10 let num2=10 console.log(num1+num2) //20 Numbers are added |
The following code is very similar to the code above. Here instead of adding two numbers, we add two strings using the +
operator. Here interpreter correctly identifies the data type as a string and hence joins them.
1 2 3 4 5 | let str1="Hello" let str2="world" console.log(str1+ str2) //HelloWorld Strings are joined |
How does the interpreter know when to perform the addition and when to join them?. By examining the data type. If both the variables are numbers then the interpreter adds them. If any one of them is a string, then it joins them.
Hence it is very important for the compiler or interpreter to identify the data type of the variable. Otherwise, it may end up joining numbers and adding strings, etc.
Typescript Data Types
JavaScript has eight data types. Seven primitive types and one object Data type. The primitive types are number
, string
, boolean
, bigint
, symbol
, undefined
, and null
. Everything else is an object in JavaScript.
The TypeScript Type System supports all of them and also brings its own special types. They are unknown, any, void & never.
TypeScript also provides both numeric and string-based enums. Enums allow a developer to define a set of named constants
The following diagram shows the relationship between various types. (Image from https://objectcomputing.com/resources/publications/sett/typescript-the-good-parts )
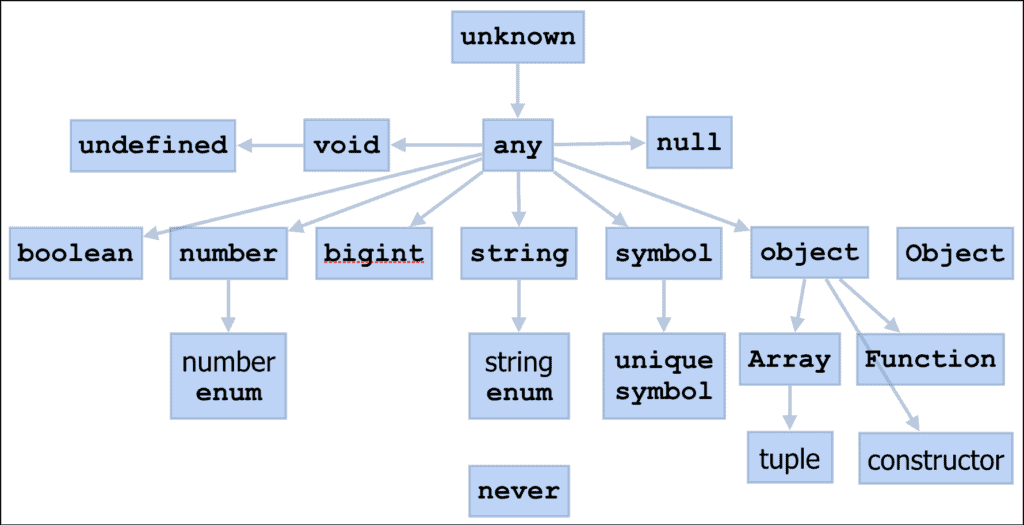
We can compose other types to create union types or intersection types. This is particularly useful when you want to combine existing types and form new ones.
TypeScript Literal Types restrict the value of a variable to finite valid values. It allows us to create String, Numeric, Boolean, and Enum Literal Types
Typescript Type Checking
TypeScript types introduce strong type checking to JavaScript. The use of types is optional, but if use the types, then they are analyzed by the compiler, and errors are thrown if they are used incorrectly. let us see it using an example.
Open Visual Studio Code and create a new file types.ts. If you are new to typescript you can refer to the tutorial Hello world example in Typescript
1 2 3 4 5 6 7 8 9 | let num1 //no type is defined. let num2 num1=100; num2=”hello” console.log(num1+num2) |
We have declared two-variable num1
& num2
. We want both variables to hold numbers. But in the subsequent line, we assign a string to num2
variable instead of a number. Now open the command window and compile and run as shown below
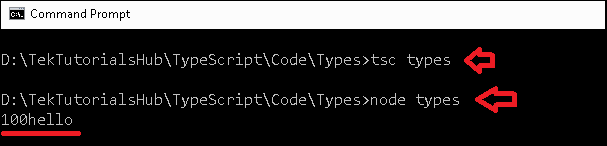
The program does not throw any errors. The result “100hello” is printed in the console as shown above
Now, modify the code and bring the Typescript Types as shown below. We are declaring that num1 & num2 will hold the type number.
1 2 3 4 5 6 7 8 9 | let num1 :number //defining type let num2 :number num1=100 num2="hello" console.log(num1+num2) |
The visual Studio code instantly underlines the code with red as soon as we use the wrong type. We also have an error thrown at us at the time of compilation as shown in the image below.
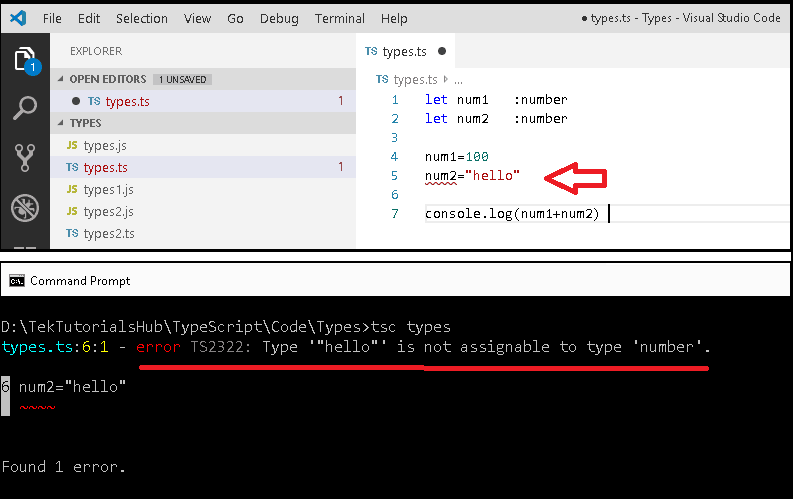
The number
is one of the many data types that Typescript supports.
Opting out of Type Checking
Any
Typescript allows us to opt-out of type checking by assigning any type to a variable. The compiler will not perform type checking on variables whose type is any.
any
is a special data type that can hold any data. You can change the data type. We use this when we do not know the type of data. any
is specific to typescript.
When a variable’s type is not given and typescript cannot infer its type from the initialization then it will be treated as an any
type.
1 2 3 4 5 6 7 8 9 10 | let notSure: any = 4; //defined as any notSure = "maybe a string instead"; notSure = false; let stillNotSure //No Type specified. inferred as any stillNotSure=5 stillNotSure="may be a strng instead" stillNotSure=false; |
You can refer to the tutorial Any Type to learn more about them.
TypeScript Special Types
Apart from any type, typescript has three other special types. They are never
, void
& unknown
types
Never
The never
type represents the value that will never happen. We use it as the return type of a function, which does not return a value. For example, the function that always throws an exception is shown below.
1 2 3 4 5 | function doSomething(): never { throw new Error("Error has occurred"); } |
You can refer to the TypeScript Never Type tutorial.
Void
Void represents the absence of any return value. For example, the following function prints “hello” and returns without returning a value. Hence the return type is void.
It is different from never. never means it never returns a value.
1 2 3 4 5 | function sayHello(): void { console.log('Hello!') } |
Unknown Type
unknown
type means that the type of variable is not known. It is the type-safe counterpart of any.
You can assign anything to an unknown type.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | let unknownVar:unknown; unknownVar = true; //boolean unknownVar = 10; //number unknownVar = 10n; //BigInt >>=ES2020 unknownVar = "Hello World"; //String unknownVar = ["1","2","3","4"] //Array unknownVar = {firstName:'', lastName:''}; // Object unknownVar = null; // null unknownVar = undefined; // undefined unknownVar = Symbol("key"); // Symbol |
But cannot assign unknown to any other types.
1 2 3 4 5 6 7 8 9 10 | let value: unknown; let value1: boolean = value; // Error let value2: number = value; // Error let value3: string = value; // Error let value4: object = value; // Error let value5: any[] = value; // Error let value6: Function = value; // Error |
Primitive Types
TypeScript supports 7 primitive types number
, string
, boolean
, bigint
, symbol
, undefined
, and null
. All other data types are objects in Typescript. A primitive data type is a data type that is not an object and has no methods. All primitives are immutable.
String
We use the string
data type to store textual data. The string value is enclosed in double-quotes (“) or single quotes (‘).
Example
1 2 3 4 | let message=”Hello World” let color=”red" |
Multiline string
The strings can span multiple lines in such cases the strings are surrounded by the backtick/backquote (`) character
1 2 3 4 | let sentence: string =`Hello, welcome to the world of typescript, the typed super of javascript` |
You can read more about string data type from the following tutorials
Boolean
The Boolean Data Type is a simple true/false value
Example
1 2 3 | let isDone: boolean = false; |
Read more about Boolean Data Type
Number
The number
data types in TypeScript are 64-bit floating-point values and are used to represent integers and fractions. Typescript also supports hexadecimal and decimal literals. It also supports the binary and octal literals introduced in ECMAScript 2015.
1 2 3 4 5 6 | let decimal: number = 10; let hex: number = 0xa00d; //hexadecimal number starts with 0x let binary: number = 0b1010; //binary number starts with 0b let octal: number = 0o633; //octal number starts with 0c |
You can read more about the number data type from the following Tutorials
Bigint
bigint
is the new introduction in Typescript 3.2. This will provide a way to represent whole numbers larger than 253. You can get a bigint
by calling the BigInt()
function or by writing out a BigInt literal by adding an n
to the end of any integer numeric literal as shown below.
1 2 3 4 | let big1: bigint = BigInt(100); // the BigInt function let big2: bigint = 100n; // a BigInt literal. end with n |
You can use the bigint only if you are targeting version ESNext.
Refer to the following tutorial to learn more about BigInt
Null and Undefined
JavaScript has two ways to refer to the null. They are null
and undefined
and are two separate data types in Typescript as well. The null
and undefined
are subtypes of all other types. That means you can assign null
and undefined
to something like a number
.
1 2 3 4 | let u: undefined = undefined; let n: null = null; |
undefined Denotes value is given to all uninitialized variables.
null: Represents the intentional absence of object value.
Refer to the Typescript Null Vs Undefined tutorial to learn more about them
Symbol
The symbol is the new primitive type introduced in ES6 and represents the javaScript symbol primitive type. it represents unique tokens that may be used as keys for object properties. it is created by the global Symbol()
function. Each time the Symbol()
function is called, a new unique symbol
is returned.
TypeScript Objects
Everything that isn’t a Primitive type in TypeScript is a subclass of the object type. Objects provide a way to group several values into a single value. You can group any values of other types like string, number, booleans, dates, arrays, etc. An object can also contain other objects. We can easily build a more complex structure using them
A Typescript object is a collection of key-value pairs. Each key-value pair is known as a property, where the key
is the name of the property and value
its value.
The following is one of the ways to create an object in TypeScript. It consists of a list of key-value pairs, each separated by a comma and wrapped inside curly braces
1 2 3 4 5 6 7 8 9 10 | let person = { firstName: "Allie", lastName: "Grater", age: 50, fullName: function() { return this.firstName + " " + this.lastName; } } |
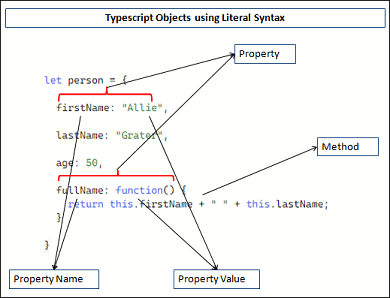
We will cover these in detail in the subsequent chapters
Summary
Using types we define the data type of variables. If we did not specify the type, then typescript treats it as any type. Any type is the base type of all other types. The primitive types are number, string, bigint, boolean, null, and undefined. All other types are derived from the objects.
Best Explanation
good