This Angular Tutorial helps you to learn the concepts of Angular. Out step by step guides walk you through building Angular Applications, and adding Components, Directives, Pipes, etc. Learn how to organize Applications using Modules, Navigate Using Routers, etc. The tutorial also covers advanced topics like component communications, Services, Forms, routers, HTTP communications, observable, SEO, etc. This Tutorial takes a simple, step-by-step approach and includes many examples and codes.
This Tutorial covers all versions of Angular, Starting from Angular 2 to the latest editions, i.e., Angular 17.
Table of Contents
- What is Angular
- Angular Versions
- Prerequisites
- Angular Tutorial
- Introduction to Angular
- Getting Started With Angular
- Components
- Directives
- Pipes
- Component Communication
- Component Life Cycle Hook
- Angular Forms
- Services & Dependency Injection
- Angular Forms Validation
- HTTP
- Angular Router
- Angular Route Guards
- Angular Module
- Advanced Components
- Observable in Angular
- Styling the Application
- Others
- Configuration
- Handling Errors
- Angular CLI
- SEO & Angular
- Angular Universal
- Building & Hosting
- Angular Resources
- Angular how-to guides
- Module Loaders
What is Angular
Angular is a Javascript Framework-based development platform for building a single-page application for mobile and desktop. It uses Typescript & HTML to build Apps. The Angular itself is written using Typescript. It now comes with every feature you need to develop a complex and sophisticated web or mobile application. Angular is loaded with features like components, Directives, Forms, Pipes, HTTP Services, Dependency Injection, etc.
Angular Versions
The early version of Angular was named Angular 2. Then later, it was renamed to just Angular. You can learn about Angular Versions
Prerequisites
This tutorial requires a working knowledge of Javascript, TypeScript, HTML & CSS. It also requires the concept of OOP.
We are going to use Typescript as our language. You will find it very easy if you know C# or Java. You can learn Typescript from the Typescript Tutorial.
Angular Tutorial
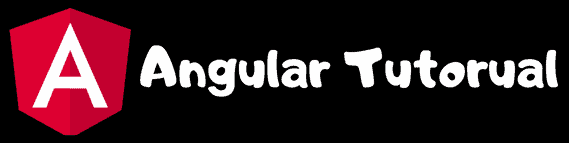
Introduction to Angular
This Introduction to Angular gives you a glimpse of Angular. Angular is a Javascript framework for building mobile and desktop web applications. It is built using Javascript. You can build amazing client-side applications using HTML, CSS, and Typescript using Angular. Knowing how the Angular framework works is essential before you start using it. The following tutorials introduce you to Angular, and you will learn Angular’s architecture.
Getting Started With Angular
Angular has undergone many changes since the version of Angular 2. From the Angular 7 version, installing and creating a new Angular project has become very simple. You only need to install and Visual Studio code, NPM Package Manager & Angular CLI. Once you install the required dependencies, creating a new project is as easy as running a simple command ng new. Angular CLI takes care of the Configuration & initialization of various libraries.
You can read the following step-by-step tutorial to learn how to create an Angular application and get started.
Components
The Component is the main building block of an Angular Application. A Component contains the definition of the View and the data that defines how the View looks and behaves. The Angular Components are plain javascript classes and defined using. @component Decorator. This Decorator provides the component with the View to display & Metadata about the class.
The Component passes the data to the view using a process called Data Binding. This is done by Binding the DOM Elements to component properties. Binding can display component class property values to the user, change element styles, respond to a user event, etc.
- Introduction to Angular Components
- Data Binding
- Interpolation in Angular
- Property Binding
- Event Binding
- Two-way Binding & ngModel
- ngModelChange & Change Event
- Adding Child Component
- Standalone Components
Directives
The Angular directive helps us to manipulate the DOM. You can change a DOM element’s appearance, behavior, or layout using the directives. They help you to extend HTML. The Angular directives are classified into three categories based on how they behave. They are Component, Structural, and Attribute Directives
The ngFor
is an Angular structural directive that repeats a portion of the HTML template once per each item from an iterable list (Collection). The ngSwitch allows us to Add/Remove DOM elements. It is similar to the switch statement of Javascript. The ngIf allows us to Add/Remove DOM elements.
The ngClass Directive is an Angular Attribute Directive, which allows us to add or remove CSS classes to an HTML element. The ngStyle directive allows you to modify the style of an HTML element using the expression. Using the ngStyle, you can dynamically change the style of your HTML element.
Pipes
The Angular pipes are used to Transform the Data. For Example, the Date pipe formats the date according to locale rules. We can pass arguments to pipe and chain pipes. Angular also allows us to create a Custom Pipe.
- Angular Pipes
- Angular Custom Pipes
- Date Pipe
- Async Pipe
- KeyValue Pipe
- Using Pipes in Components & Services
Component Communication
- Angular Component Communication
- Passing data from Parent to child component
- Passing Data from Child to Parent Component
Component Life Cycle Hook
The life cycle hooks are the methods that angular invokes on directives and components as it creates, changes, and destroys them. Using life-cycle hooks, we can fine-tune the behavior of our components during creation, update, and destruction.
Angular Forms
The data entry forms can be very simple to very complex. The Forms contain a large no of input fields, a variety of fields like Text boxes, Dates, Numbers, Emails, Passwords, Check Boxes, Option boxes, etc. These fields can Span multiple tabs or multiple pages. Forms may also contain complex validation logic interdependent on multiple fields.
The Angular forms modules are designed to handle the above and more. Angular Forms now supports the Reactive forms approach to Forms development. The older way of Template-based approach is also supported.
- Angular Forms Tutorial: Fundamental & Concepts
- Template-Driven Forms in Angular
- Set Value in Template Driven forms in Angular
- Reactive Forms in Angular
- FormBuilder in Reactive Forms
- SetValue & PatchValue in Angular
- StatusChanges in Angular Forms
- ValueChanges in Angular Forms
- FormControl
- FormGroup
- FormArray Example
- Build Dynamic or Nested Forms using FormArray
- SetValue & PatchValue in FormArray
- Select Options Dropdown
- Typed Forms in Angular
- FormRecord in Angular
Services & Dependency Injection
Services allow us to create reusable code and use it for every component that needs it. The Services can be injected into components and other services using the dependency injection system. The dependencies are declared in the Module using the Provider’s metadata. The Angular creates a tree of injectors & Providers that resembles the Component Tree. This is called the hierarchical pattern.
- Services
- Dependency injection
- Injector, @Injectable & @Inject
- Providers
- Injection Token
- Hierarchical Dependency Injection
- Angular Singleton Service
- ProvidedIn root, any & platform
- @Self, @SkipSelf & @Optional Decorators
- @Host Decorator in Angular
- ViewProviders
Angular Forms Validation
One of the common tasks that is performed while building a form is Validation. The Forms Validation is built into the Angular Forms Module. Angular provides several Built-in validators out of the box. If those validators do not fit your needs, you can create your own custom validator.
- Validations in Reactive Forms in Angular
- Custom Validator in Reactive Forms
- Passing Parameter to Custom Validator in Reactive Forms
- Inject Service into Custom Validator
- Validation in Template-Driven Forms
- Custom Validator in Template-Driven Forms
- Angular Async Validator
- Cross Field Validation
- Adding Validators Using SetValidators
HTTP
The newly designed HttpClient Module allows us to query the Remote API source to get data into our Application. It requires us to Subscribe to the returned response using RxJs observables.
- Angular HTTP Client Tutorial
- HTTP GET Example
- HTTP POST Example
- Passing URL Parameters (Query strings)
- HTTP Headers Example
- HTTP Interceptor
Angular Router
The Router module handles the navigation & Routing in Angular. The Routing allows you to move from one part of the application to another part or one View to another View.
- Angular Router
- Angular Router with standalone components
- Location Strategies in Angular Router
- Passing Parameters to Route
- Child Routes / Nested Routes
- Query Parameters
- Navigation between Routes
- Angular Pass data to route
- RouterLinkActive
- Router Events
- ActivatedRoute
Angular Route Guards
Angular Module
The Angular Modules help us to organize our code into manageable parts or blocks. Each block implements a specific feature. The Components, Templates, Directives, Pipes, and Services that implement that feature become part of the module. The following tutorial explains how best you can create an Angular Module, The folder structure you can use, etc. We can also load the Modules lazily or Preload them, thus improving the application’s performance.
- Introduction to Angular Modules
- Routing Between Angular Modules
- Angular folder structure: Best Practices
- Lazy Loading in Angular
- Preloading Strategy
- CanLoad Guard
Advanced Components
The Components in Angular are very powerful features. The following tutorials take you through some of the important features of the Angular Component.
- Ng-Content & Content Projection in Angular
- Angular @input, @output & EventEmitter
- Template Reference Variable in Angular
- ng-container in Angular
- ng-template & TemplateRef in angular
- ngtemplateoutlet in angular
- HostBinding & HostListener
- ViewChild, ViewChildren & QueryList
- ElementRef
- Renderer2
- ContentChild & ContentChildren
- AfterViewInit, AfterViewChecked, AfterContentInit & AfterContentChecked
- Angular Decorators
Observable in Angular
Angular uses the Observable Pattern extensively. The following tutorials give you an introduction to observable and how to use it in an Angular Application.
- Angular Observable Tutorial
- Create an Observable from a string, array, object, collection
- Observable from events using fromEvent
- Observable pipe
- Map Operator
- Filter Operator
- Tap Operator
- SwitchMap
- MergeMap
- ConcatMap
- ExhaustMap
- take, takeUntil, takeWhile, takeLast
- First, last & Single
- Skip, SkipWhile, SkipUntil & SkipLast
- Scan & Reduce
- DebounceTime & Debounce
- Delay & DelayWhen
- ThrowError
- CatchError
- Retry & ReTryWhen
- Unsubscribe from an observable
- Subjects in Angular
- ReplaySubject, BehaviorSubject & AsyncSubject
- Angular Subject Example
Styling the Application
Angular uses several different ways to style the Application. You can style the app globally and then override it locally in the component very easily. The component styles have local scope, which is achieved using the various View Encapsulation strategies. Learn all these in the section
- Angular Global Styles
- View Encapsulation
- Style binding in Angular
- Class Binding in Angular
- Component Styles
- How to Install & Use Angular FontAwesome
- How to Add Bootstrap to Angular
Others
Configuration
The apps usually need some Run-time configuration information like URL endpoint etc., which it needs to load at startup. Also, different environments like development, production & testing require different environments, etc.
Handling Errors
In the following Angular tutorials, we look at how Angular handles errors. We handle errors by setting up a Global Error handler or custom error handler. Also,.whenever the error occurs in an HTTP operation, Angular wraps it in an httpErrorResponse
Object. Learn how to handle HTTP Errors also.
Angular CLI
Learn how to use Angular CLI to speed up the development of Angular Application
- Angular CLI Tutorial
- ng new
- Upgrading Angular App to the latest version
- Migrating to Standalone Components
- Multiple Apps in One Angular Project
SEO & Angular
Your Website is useless if the Search Engines do not find it. There are many things you must consider to make your App SEO-friendly. You need to set up Title & Meta Tags for each page. Ensure that the search engines can crawl and read your page. Set the correct Canonical URL for each page etc. Also, ensure that the app loads quickly, etc. The Following Angular Tutorials guide you through some of the important SEO features.
- Title Service Example
- Dynamic Title based on Route
- Meta Service
- Dynamic Meta Tags
- Canonical URL
- Lazy Load Images in Angular
Angular Universal
The following Angular Universal Tutorial explains how to achieve Server Side Rendering using Angular Universal. The App’s rendering on the server side makes it load quickly and ensures that the search engines can crawl the content.
Building & Hosting
Angular Resources
Angular how-to guides
- How to get the current Route
- ExpressionChangedAfterItHasBeenCheckedError in Angular
- Angular CLI Check Version
- Property ‘value’ does not exist on type ‘EventTarget’ Error in Angular
Module Loaders
The Angular application can use either SystemJs or Webpack module loader. We will demonstrate how to use both loaders by building a small application.
Great tutorial! I’m excited to dive into learning Angular after being a Hub reader for a while now. Your explanations are clear and easy to follow, and I appreciate the examples and links provided throughout the post. Thanks for sharing your knowledge with the community!
Thanks
your tutorials about angular are amazing
can you update to Angular 17
++1
Thanks a lot. You have outstanding teaching skills. It’s exactly what I needed.
Awesome Content, the best explanation and examples
Thanks for examples
Awesome Content, best explanation and examples
amazing and kudos !!
awaiting for your upcoming tutorials on React , Node and Python 😇
Content wise super presentation and coverage.
Please upload angular localization topic.
The Best Content ever …!
very structured documentation with easy words to understand complex concepts
Can you please ad component and service test cases implementation.
sure
Excellent !
can you please add promise tutorial
Superb Course Mind Blowing Hat’s Off 🙏
Hi tektutorialshub,
Could you please share link promises for Angular observable?
This is very nice and properly explained tutorial. Just a suggestion, can we please have all detailed index for topics. Like when we click on right and left arrow for navigation which topic and subtopic will come, if that we can get in index page on Angular tutorial home page it will be helpful. Thank you for your great work.
Nice tutorials on Angular.
Good tutorial. Thanks for sharing this to us.
You are the best to explain concepts
I’m addict to this website
Thanks
The best angular content I ever seen in my 5-year work experience
The best tutorial ever seen in my Angular study.
I didn’t find any website better than this.
How well structured and well self explainary this course is.
ekdam sachi vat…
Satya Vachan
extremely excellent. Thank u so much my friend!
Very good content of angular i found on net. Nicely and easily explained each thing.
Hello Admin,
So far, this is one of the best content and most easy way tutorial I have found on net. Is there any PDF available for this tutorial? I really want to print it and study it.
Best tutorial easy to understand and given excellent exaamples
Hi. This tutorial is great! Its easy to understand.
Please put tutorial on node.js and React
Please provide interview questions angular
Please provide Node Js tutorial
is there any changes in angular 13?? These docs are valid for version 13??
As long as I am studying it, looks like it is pretty much the same.
Excellent!
Excellent tutorial & very well explained!
Great website for beginner,
Extremely good tutorial! Thank you!
Great! very simple. Beginner to advanced level
Great! very simple. Beginner to advanced.
best site for learn angular —fresher
The best of the best!!
Excellent site to learn angular.
I bookmarked it when I need good information about the ngModule annotation, when I started my work on angular, I started going through this tutorial.
It is really helping me to understand very well about the angular.
Thank you very much for sharing the knowledge in a better crafted story to grab the knowledge very well.
ytddytd
Great!!
Excellent Tutorial , Many Thanks for sharing the knowledge.
God bless you!
Best site to learn Angular. Nicely explained and easy to understand.
Best Tutorial in Web for Angular.
No any tutorial can be better from this one. Thanks a lot !
This is the best angular tutorial which i have ever read.simply and understandable keep it up.
Can i get this entire tutorial in PDF format ?
Same request, I would be happy to pay for it. Thanks!
Easily Understandable. Thanks, Can you please provide a good example of making complete app from start to end like: Step by step of making Header, Navigation, Content area and Footer so that one can easily make a app after seeing that.